I have problem with my atapi driver. It is return 0 forever in qemu and Bochs and 65535 in Virtualbox. Please where is bug?
Code: Select all
void atapi_read(uint16_t byte_count, uint32_t sector) {
//select drive master
outb(0x176, 0xE0);
//Use PIO
outb(0x171, 0);
//Maximal byte count
outb(0x174, (byte_count & 0xff));
outb(0x175, (byte_count >> 8));
//ATAPI packet command
outb (0x177, 0xA0);
tp("debug 1");
//Wait
while( (inb(0x177) & 0x88)!=0x08 ) {}
//Command set
outw(0x170, 0xA8);
outw(0x170, 0);
outw(0x170, (unsigned char)(sector >> 24));
outw(0x170, (unsigned char)(sector >> 16));
outw(0x170, (unsigned char)(sector >> 8));
outw(0x170, (unsigned char)(sector >> 0));
tp("debug 2");
//Wait for irq
while( ide_secondary_interrupt==0 ) {}
//Read lenght of input/output
uint16_t size = ( ((unsigned short)(inb(0x175) << 8)) | ((unsigned short)inb(0x174)) );
uint16_t lenght = (size / 2);
tp_var(size);
//Write or read data
for(int i=0; i<lenght; i++) {
cdrom_buffer[i]=inw(0x170);
}
tp("debug 3");
}
Code: Select all
00095487733i[HD ] READ(12) with transfer length <= 0, ok (0)
00095488831e[HD ] IO read(0x0170) with drq == 0: last command was a0h
...
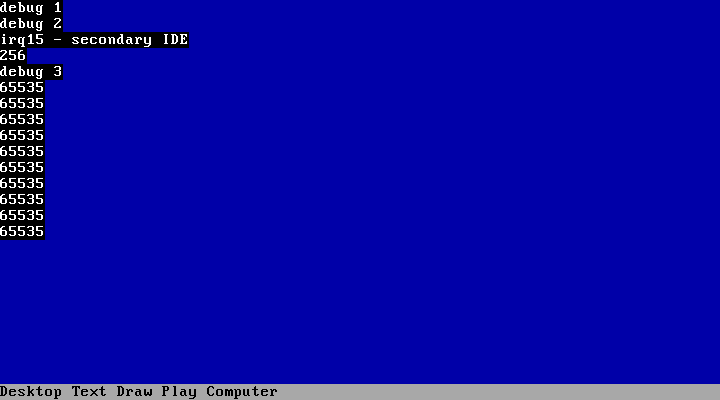